Từ A-Z về Axios. Hướng dẫn kiểm soát lỗi khi request HTTP với Axios
Axios là thư viện HTTP mạnh mẽ giúp gửi và xử lý request nhanh chóng trong JavaScript. Khám phá cách dùng Axios hiệu quả trong phát triển web hiện đại.
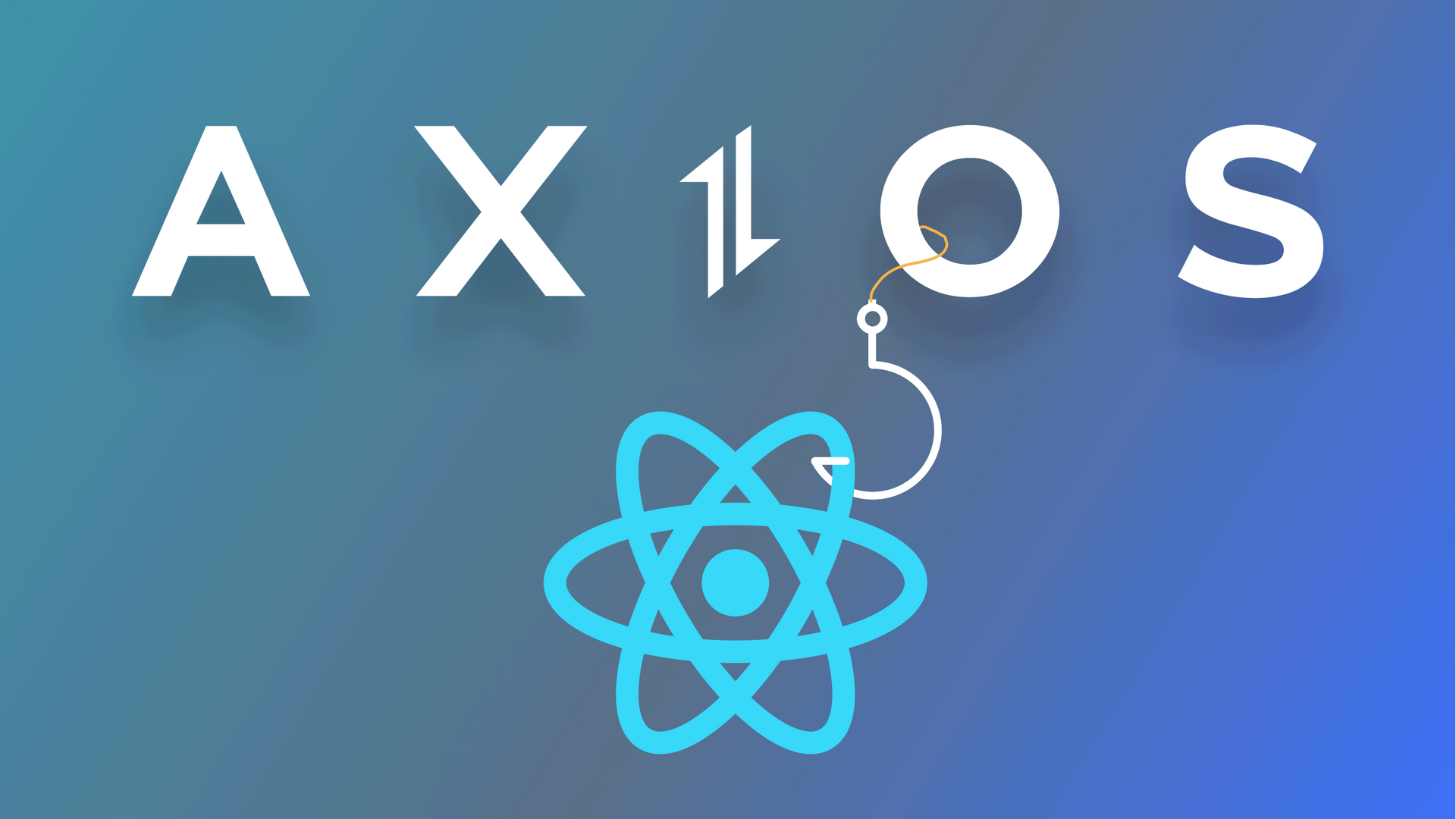
- Axios là gì?
- Các tính năng của Axios
- Một số phương thức của Axios
- Gửi yêu cầu GET
- Gửi yêu cầu POST
- Gửi nhiều yêu cầu cùng lúc
- Cấu hình nâng cao khi gửi request với Axios
- Cấu trúc dữ liệu response trong Axios
- Kiểm soát lỗi khi request HTTP với Axios
- Hủy bỏ yêu cầu
- Hook API (Interceptor)
- Ví dụ về ứng dụng với Axios
- Lời kết
Axios là gì?
Axios là một thư viện HTTP client trong Javascript được thiết kế dựa trên đối tượng Promise. Công cụ này thường được sử dụng phổ biến trong các ứng dụng frontend như Angular, React hay Vue.js… Với Axios, người dùng có thể dễ dàng gửi đi các request HTTP bất đồng bộ như GET, POST, DELETE, PUT đến các REST endpoint và thực hiện các chức năng CRUD đơn giản và linh hoạt.
Không chỉ thế, Axios còn cung cấp nhiều tính năng hữu ích khác như:
- Interceptor: Hỗ trợ can thiệp vào quá trình gửi và nhận yêu cầu
- Transform data: Cho phép thao tác với dữ liệu trước khi gửi và nhận
- XSRF protection: Bảo vệ ứng dụng trước rủi ro từ các cuộc tấn công XSRF
Các tính năng của Axios
Một số tính năng chính của Axios bao gồm:
- Gửi request từ trình duyệt bằng XMLHttpRequest
- Gửi request trong Node.js bằng HTTP
- Hỗ trợ Promise API
- Can thiệp vào request/response qua interceptor
- Biến đổi dữ liệu trước/sau khi gửi/nhận
- Hủy yêu cầu HTTP với cancel token
- Tự động chuyển đổi dữ liệu sang JSON
- Bảo vệ khỏi tấn công XSRF
Một số phương thức của Axios
Gửi yêu cầu GET
axios.get('http://demo.com/user?ID=12345') .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
Hoặc dùng params
:
axios.get('http://demo.com/user', { params: { ID: 12345 } }) .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
Gửi yêu cầu POST
axios.post('http://demo.com/user', { name: 'TuandungB', email: 'tuandungb@demo.com', password: '123456' }) .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); });
Gửi nhiều yêu cầu cùng lúc
function getAccountInfomation() { return axios.get('/account/12345'); } function getOrderInfomation() { return axios.get('/order/12345'); } axios.all([getAccountInfomation(), getOrderInfomation()]) .then(axios.spread(function (account, order) { // Cả hai yêu cầu hoàn tất }));
Cấu hình nâng cao khi gửi request với Axios
Bạn có thể cấu hình nâng cao cho mỗi request bằng cú pháp:
axios(url[, config])
Ví dụ đối tượng config
:
{ url: '/user', method: 'get', baseURL: 'https://demo.com/api/', transformRequest: [function (data, headers) { return data; }], transformResponse: [function (data) { return data; }], headers: {'X-Requested-With': 'XMLHttpRequest'}, params: { ID: 12345 }, data: { firstName: 'Nguyen' }, timeout: 1000, withCredentials: false, auth: { username: 'tuandungb', password: '123456' }, responseType: 'json', xsrfCookieName: 'XSRF-TOKEN', xsrfHeaderName: 'X-XSRF-TOKEN', onUploadProgress: function (progressEvent) {}, onDownloadProgress: function (progressEvent) {}, maxContentLength: 2000, validateStatus: function (status) { return status >= 200 && status < 300; }, maxRedirects: 5, httpAgent: new http.Agent({ keepAlive: true }), httpsAgent: new https.Agent({ keepAlive: true }), proxy: { host: '127.0.0.1', port: 9000, auth: { username: 'tuandungb', password: '123456' } }, cancelToken: new CancelToken(function (cancel) {}) }
Cấu trúc dữ liệu response trong Axios
Một response từ server thông qua Axios thường có dạng:
{ data: {}, // Dữ liệu từ server status: 200, // Mã trạng thái HTTP statusText: 'OK', // Mô tả trạng thái headers: {}, // Header response config: {}, // Cấu hình request ban đầu request: {} // Thực thể XMLHttpRequest (trình duyệt) hoặc ClientRequest (Node.js) }
Ví dụ:
axios.get('http://demo.com/user/12345') .then(function(response) { console.log(response.data); console.log(response.status); console.log(response.statusText); console.log(response.headers); console.log(response.config); });
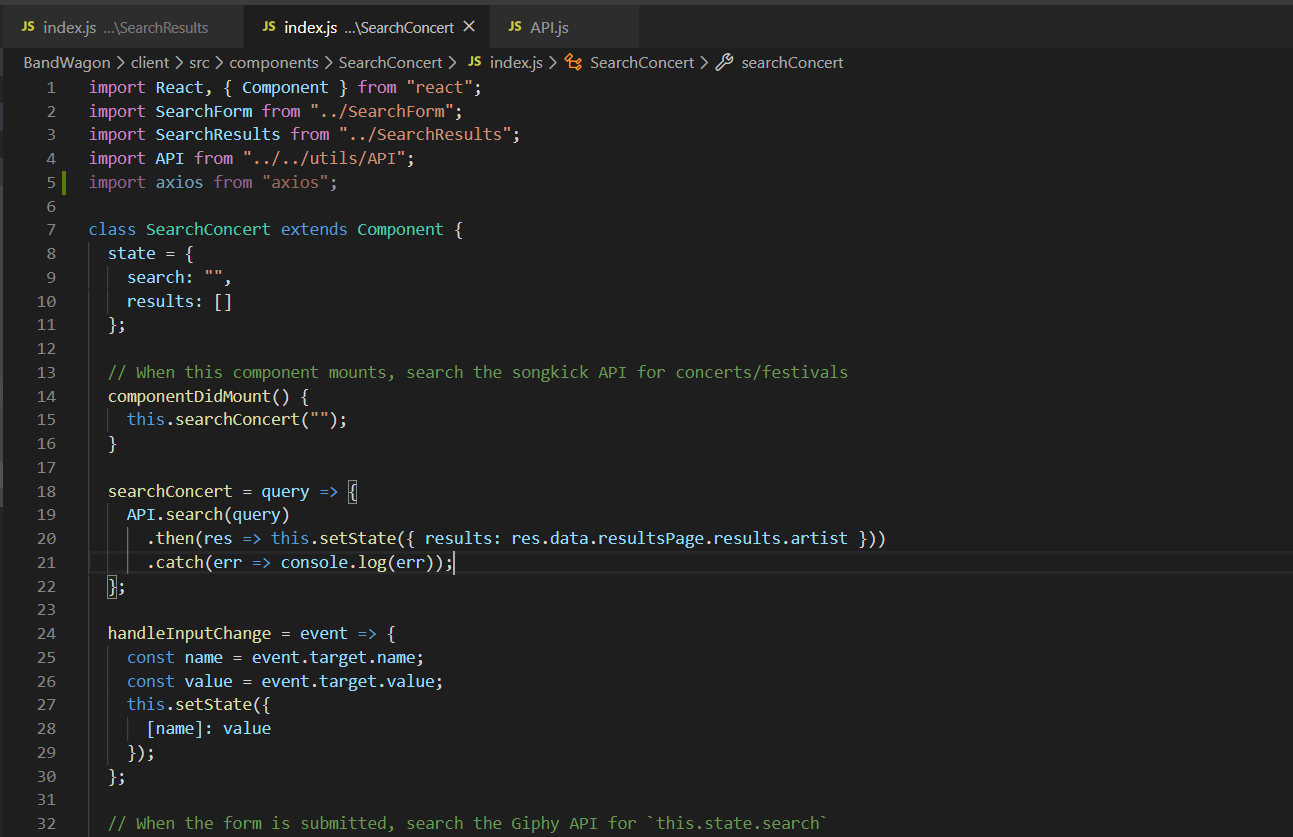
Kiểm soát lỗi khi request HTTP với Axios
Bạn có thể xử lý các lỗi khác nhau một cách linh hoạt:
axios.get('http://demo.com/user/12345') .catch(function (error) { if (error.response) { console.log(error.response.data); console.log(error.response.status); console.log(error.response.headers); } else if (error.request) { console.log(error.request); } else { console.log('Error', error.message); } console.log(error.config); });
Hủy bỏ yêu cầu
Dùng CancelToken
để hủy request nếu cần:
var CancelToken = axios.CancelToken; var source = CancelToken.source(); axios.post('http://demo.com/order', { data: { 'product_id': 234, 'quantity': 3 }, cancelToken: source.token }).catch(function(thrown) { if (axios.isCancel(thrown)) { console.log('Hủy bỏ yêu cầu', thrown.message); } else { // Xử lý lỗi } }); // Người dùng quyết định hủy yêu cầu source.cancel('Hủy yêu cầu tạo đơn hàng mới.');
Hook API (Interceptor)
Khi gửi request, bạn có thể muốn thực hiện một hành động như hiển thị tiến độ, loading bar hoặc log dữ liệu trước khi gửi hoặc sau khi nhận phản hồi. Lúc này, Interceptor trong Axios là giải pháp phù hợp.
Ví dụ kết hợp thư viện nprogress
để hiển thị tiến trình:
axios.interceptors.request.use(function (config) { NProgress.start(); // Bắt đầu thanh tiến trình return config; }, function (error) { return Promise.reject(error); }); axios.interceptors.response.use(function (response) { NProgress.done(); // Kết thúc thanh tiến trình return response; }, function (error) { return Promise.reject(error); });
Ví dụ về ứng dụng với Axios
Trong bối cảnh các ứng dụng web cần xử lý dữ liệu và hiển thị thông tin từ API nhanh chóng, Axios là lựa chọn tối ưu vì:
- Cú pháp ngắn gọn hơn Fetch API
- Hỗ trợ nhiều tính năng nâng cao như interceptors, transform data
- Dễ dùng và xử lý bất đồng bộ hiệu quả
Ví dụ lấy dữ liệu tin tức mới nhất từ API VnExpress:
axios.get('https://api.vnexpress.net/rss/tin-moi-nhat.rss') .then(response => { const data = response.data; // Xử lý dữ liệu RSS và hiển thị lên giao diện }) .catch(error => { console.error('Lỗi khi lấy dữ liệu:', error); });
Lời kết
Bài viết đã giúp bạn hiểu rõ Axios là gì, cách sử dụng các tính năng như gửi request, cấu hình nâng cao, xử lý lỗi, hủy bỏ yêu cầu và xây dựng ứng dụng thực tế. Axios là công cụ cực kỳ hữu ích cho mọi lập trình viên web hiện đại khi làm việc với HTTP request.